distance_calculator
The distance calculator is a module for calculating distance between two geographic locations. It measures the distance along the surface of the earth. Formula takes into consideration the radius of the earth. For this algorithm, it is necessary to define an object that has longitude and latitude properties like this:
(location:Location {lat: 44.1194, lng: 15.2314})
Trait | Value |
---|---|
Module type | module |
Implementation | C++ |
Graph direction | undirected |
Edge weights | unweighted |
Parallelism | sequential |
Procedures
info
If you want to execute this algorithm on graph projections, subgraphs or portions of the graph, be sure to check out the guide on How to run a MAGE module on subgraphs.
single(start, end, metrics, decimals)
Input:
start: Vertex
➡ Starting point to measure distance. Required to have lng and lat properties.end: Vertex
➡ Ending point to measure distance. Required to have lng and lat properties.metrics: string
➡ Can be either "m" or "km". These stand for meters and kilometers respectively.decimals:int
➡ Number of decimals on which you want to round up number.
Output:
distance: double
➡ The final result obtained by calculating distance (in 'm' or 'km') between the 2 points that each have its latitude and longitude properties.
Usage:
MATCH (n:Location), (m:Location)
CALL distance_calculator.single(m, n, 'km')
YIELD distance
RETURN distance;
multiple(start_points, end_points, metrics, decimals)
Input:
start_points: List[Vertex]
➡ Starting points to measure distance collected in a list. Required to have lng and lat properties. Must be of the same size as end_points.end_points: List[Vertex]
➡ Ending points to measure distance collected in a list. Required to have lng and lat properties. Must be of the same size as start_points.metrics: string
➡ Can be either "m" or "km". These stand for metres and kilometres respectively.decimals:int
➡ Number of decimals on which you want to round up number.
Output:
distance: List[double]
➡ The final result obtained by calculating distance (in meters) between the 2 points who each have its latitude and longitude.
Usage:
MATCH (n), (m)
WITH COLLECT(n) AS location_set1, COLLECT(m) AS location_set2
CALL distance_calculator.multiple(location_set1, location_set2, 'km') YIELD distances
RETURN distances;
Example
- Step 1: Input graph
- Step 2: Cypher load commands
- Step 3: Running command
- Step 4: Results
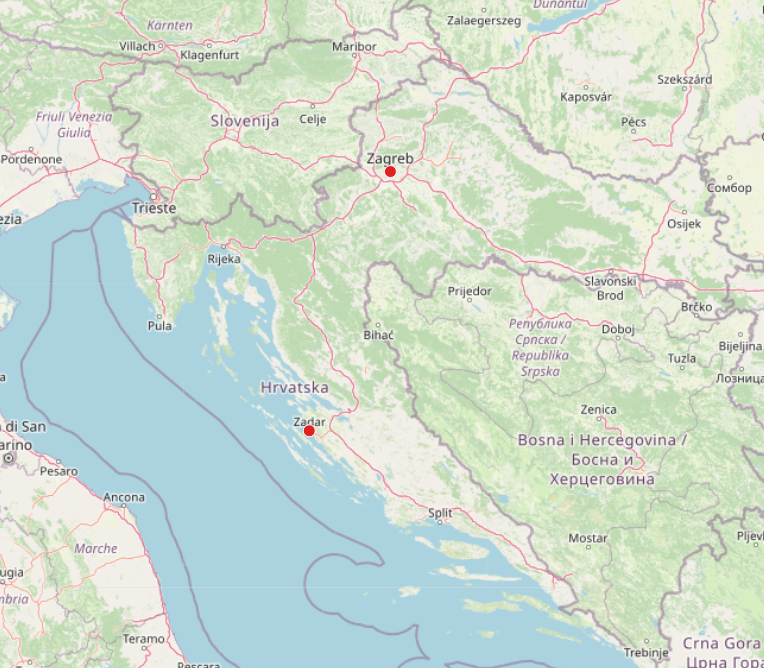
CREATE (location:Location {name: 'Zagreb', lat: 45.8150, lng: 15.9819});
CREATE (location:Location {name: 'Zadar', lat: 44.1194, lng: 15.2314});
MATCH (n {name: 'Zagreb'}), (m {name: 'Zadar'})
CALL distance_calculator.single(n, m, 'km') YIELD distance
RETURN distance;
+----------+
| distance |
+----------+
| 197.568 |
+----------+